How's the development going, @Luno? Any news you can share?
Hey Moch, thanks for following up. I'd sort been keeping quiet since no one had really asked, but I guess now's as good a time as any to say that I ended up pausing development on Year In The Trees (a giant-sized game) to make a different, medium-sized game that I can actually finish solo. I've been working on this new game pretty frantically in the mean time. I'm going to do a much longer post about this when I'm ready to announce the new game relatively soon (keyword relatively).
To make a long story short though, I very nearly had funding in hand from a major pub but the deal ended up being cancelled (just kinda fell through, there was no drama or anything). After that, I spoke with some other publishers but it wasn't really going anywhere. I decided that maybe I should try Kickstarter and started working on a trailer...I actually planned to announce by changing the name of the game and even bought the domain and built a new website!
In the middle of creating material for the Kickstarter though, I had a number of personal setbacks and had to face up to the hard fact that even the smallest version of this game was not gonna be possible for me to finish solo nights and weekends. Amidst increasing health problems (not related to burn out per se), there was also about a 0% chance a Kickstarter campaign could cover my health care costs and let me quit my job.
Needless to say, having to start over hit me pretty hard and there was a lot of emotional turmoil that I'll get into in another post. With the clarity of hindsight now though, it was absolutely the right decision; working on a game I can actually finish and release is honestly probably the best thing I can do to put me in a position where I can complete Year In The Trees some day.
So after a few months of tinkering, I was able to pick myself back up and I hit the ground running on a promising new idea using everything I've learned over the last however many years. I've posted a few vague art assets on Twitter, and I'll share those here too:
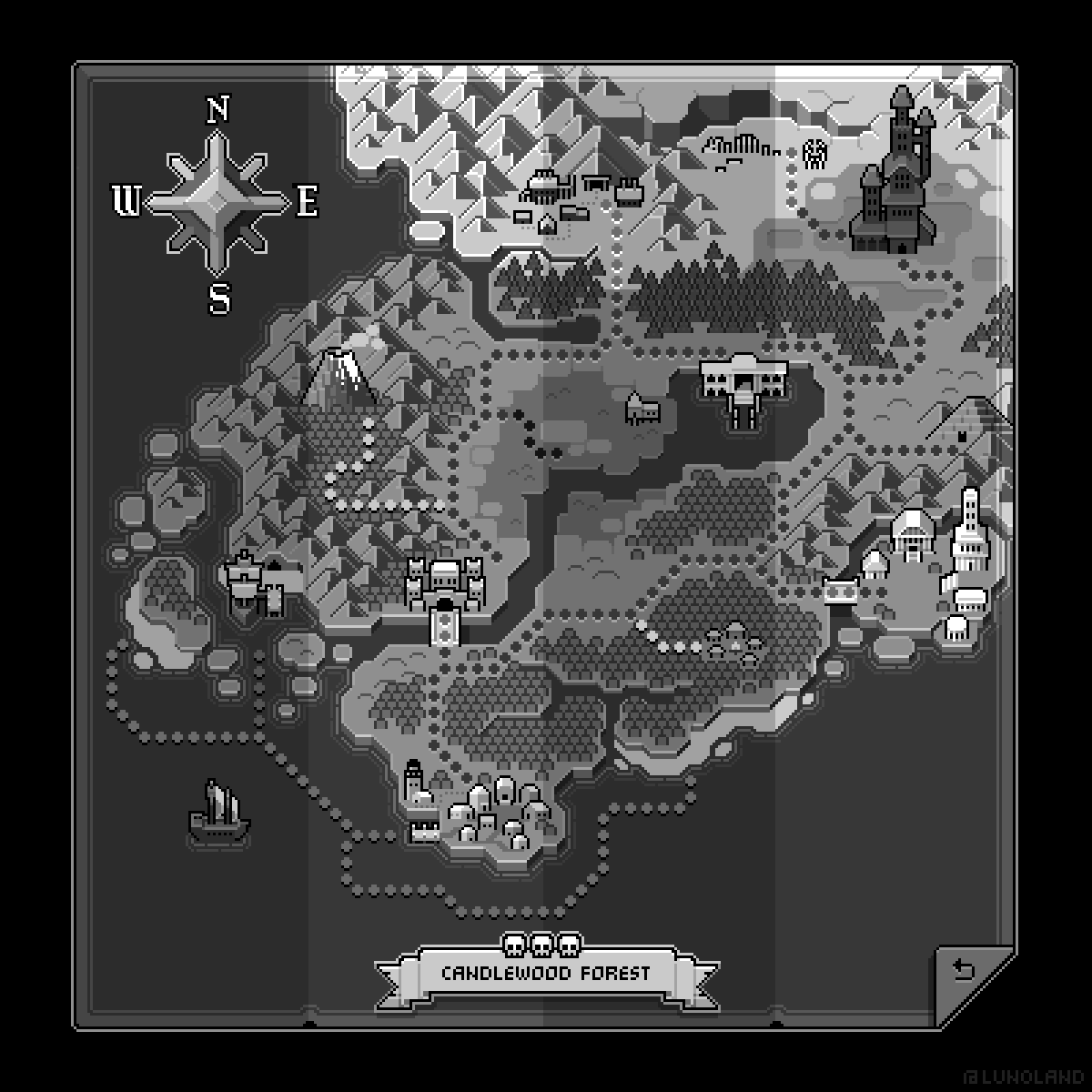
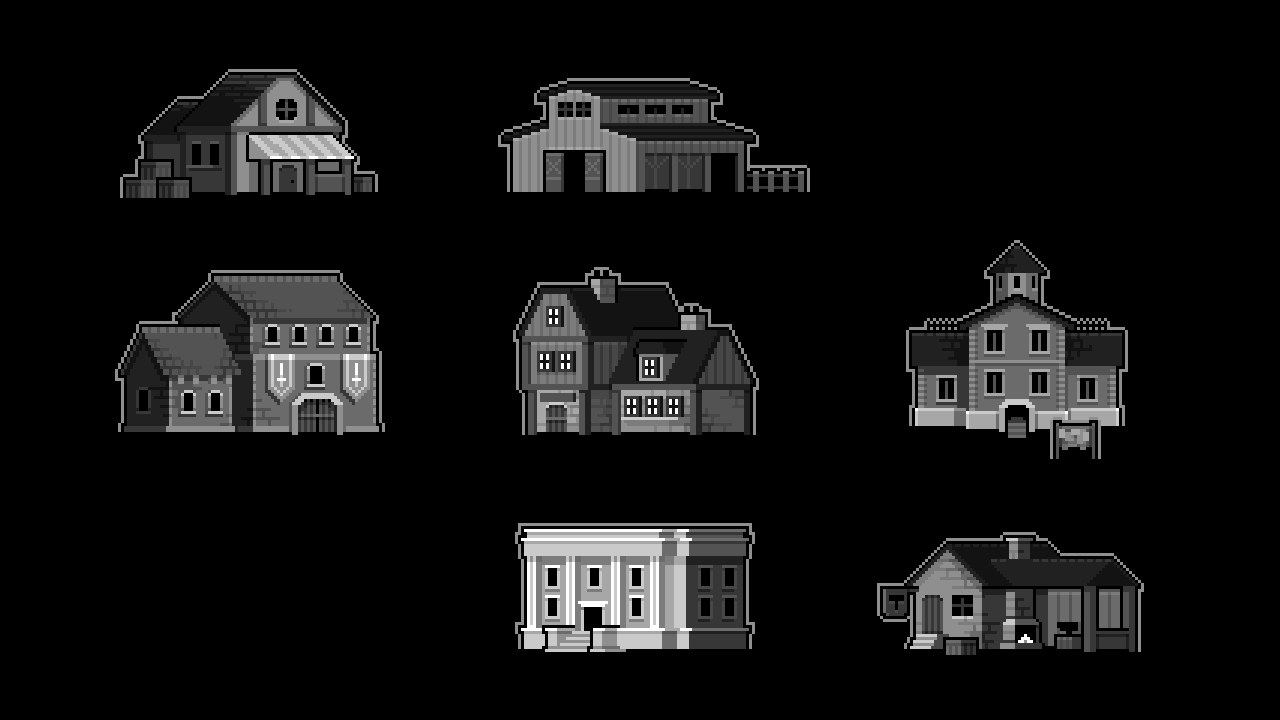
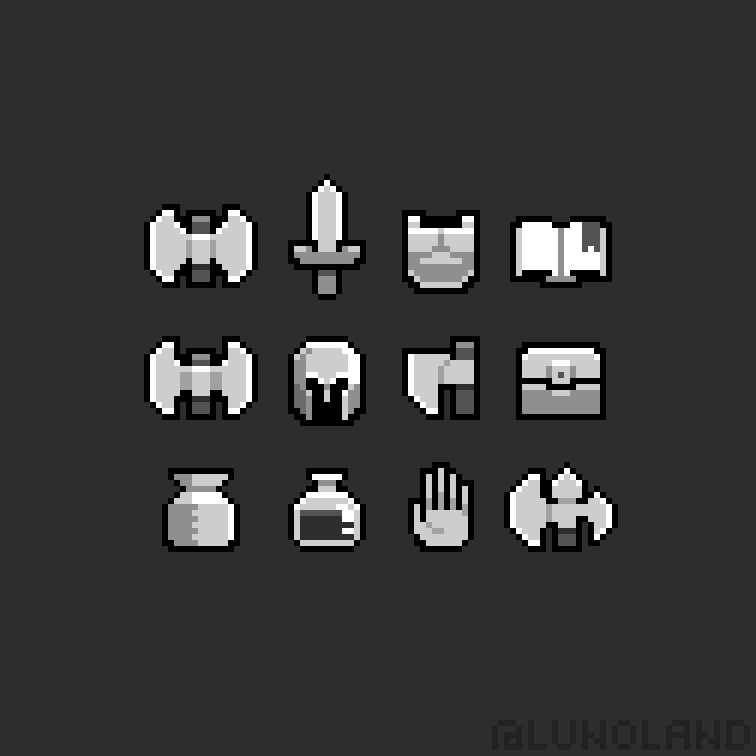

I've decided to keep this project a secret so I haven't published any footage of the prototypes (it started as a text-only game), but you can sometimes catch me streaming it on Twitch.
The development on this new game has been very different, but in a good way. On the previous game, I put a lot of pressure on myself to get noticed and produce something that was visually impressive.
After all that struggle I finally feel like I've proven to myself I'm capable of making a good-looking GIF, so this time around I've been free to focus on the nuts and bolts first and get off the ground much more quickly. The black & white art was a part of that too, though it will be full-color soon (I'm planning to keep that darker, NES-style look using high-contrast colors).
I've also been working with other solo devs for production guidance which has really helped me keep a laser focus on the work that matters most to finishing the game. Everything has been going relatively well, especially lately. At this point, the core loop is completely done and I've had a couple private demos that were way, way more well-received that I had hoped (even calling the gameplay "already too deep"

). Right now I'm wrapping up missing visual communication and polish, and I just finished the save system. Next I'll be working on the early game pacing and tutorial stuff.
Once that's all done I'll let anyone interested play a semi-public demo (which I will post about here), and then I will announce the game! Following that, there will be another art pass, sound, some boilerplate, a little more content, and then the first public demo will be ready (I'll probably adjust the scope again based on the reception).
I'm still pretty nervous people are going to be mad at me that I had to start a new game, or that it's just not going to be as appealing to people who were anticipating the old game since it's a different genre. Without saying too much though, to me this game almost has the feeling of playing a JRPG or something but it all takes place on a single screen and everything has been designed from the ground up to help me make content as efficiently as possible. There are already 10 enemies, 3 dungeons, and 79 (!!) abilities, woo
