gimymblert
|
 |
« Reply #900 on: October 25, 2016, 05:51:46 PM » |
|
Come to the dark side and try a linear congruential generator! Who needs any semblance of quality when you've got raw speeed!  static unsigned int seed;
void seed(int newseed) { seed = newseed; }
int roll(void) { seed = (seed * 214013 + 2531011) % 2147483648; return seed >> 16; }
Still a pattern with successive seed sorry! (it goes 3 by 3) Randomness is surprisingly serious business I need randomness across successive SEED ...
|
|
|
Logged
|
|
|
|
surt
|
 |
« Reply #901 on: October 25, 2016, 06:51:42 PM » |
|
You can combine multiple different LCGs to increase apparent randomness quality.
|
|
|
Logged
|
|
|
|
gimymblert
|
 |
« Reply #902 on: October 25, 2016, 07:39:50 PM » |
|
That won't solve the original problem though, it's that seed must be isolate from each other so that there is no relation between consecutive seed. Combining LCG don't help with that. IN general, pcg proposed are sequenced randomness they don't allow random "access". You can't compute the nth random number without computing the nth-1.
|
|
|
Logged
|
|
|
|
gimymblert
|
 |
« Reply #903 on: October 28, 2016, 07:10:09 PM » |
|
After looking at a lot of stuff I decided to go with this (if anyone is interested) for now:
(nested loop of i and j, basically x and y) width = (i*10000) + (j*100) Seed (1 + (i*width) + (j*width*width) )
Not sure if it's optimal but it work for the prototype I'm doing
|
|
|
Logged
|
|
|
|
gimymblert
|
 |
« Reply #904 on: November 03, 2016, 07:31:28 PM » |
|
I'm trying to make a small code that hash the space, generate 1 to 4 points inside, and link point inside a cell to the nearest point in the 8 neighbors ... BUT I have a small bug ... it doesn't seem to take obvious close point in neighbor's cell ...   ;loop all points to compute distance ; loop relative positions of neighbor cell For g = -1 To 1 For h = -1 To 1 ; if central cell, skip (don't link point within that cell) If g = 0 And h = 0 Then Goto label
; bound test If i+g < 0 Or j+h < 0 Then Goto label ;setting the current neighbor looped.cell = unit.cell(i+g,j+h)
;looping through all points of the current neighbor For l = 0 To looped\number loopt.point = cellpoint(looped,l)
;computing the square magnitude to compare lsx = Abs (loopt\x - pos\x) lsy = Abs (loopt\y - pos\y) distanceSqr = lsx^2 + lsy^2
;check for the lowest magnitude for this point If distanceSqr < distanceMin Then distanceMin = distanceSqr pointMin = loopt pointMin\i = g pointMin\j = h EndIf Next .label Next Next
It's blitzcode: x.type is how you declare custom type (basically struct) x\var is how you access member field of the struct ";" is to comment stuff
|
|
|
Logged
|
|
|
|
gimymblert
|
 |
« Reply #905 on: November 03, 2016, 07:54:29 PM » |
|
NEVER MIND   I WAS COMPUTING DISTANCE USING THE RELATIVE LOCAL CELL POSITION (as if they were inside the same cell) INSTEAD OF USING ABSOLUTE POSITION WRONG!!!!!!!! 
lsx = Abs(loopt\x - pos\x) lsy = Abs(loopt\y - pos\y) CORRECT!!! 
lsx = Abs ( (i*cx + g*cx + loopt\x) - (i*cx + pos\x) ) lsy = Abs ( (j*cy + h*cy + loopt\y) - (j*cy + pos\y) ) distanceSqr = lsx^2 + lsy^2
|
|
|
Logged
|
|
|
|
gimymblert
|
 |
« Reply #906 on: November 14, 2016, 01:36:48 PM » |
|
Okay, now do someone know how to make a staircase function where each step is 2^x in size?
Generally to make a stair case linear function you do something like int i = x/step, but I don't know what step should be to have power of 2 size of step :/
|
|
|
Logged
|
|
|
|
BorisTheBrave
|
 |
« Reply #907 on: November 14, 2016, 02:34:40 PM » |
|
// Which step you are on. int i = floor( log(x) / log(2) ) // Height of that step float h = pow(2, i)
|
|
|
Logged
|
|
|
|
gimymblert
|
 |
« Reply #908 on: November 14, 2016, 10:25:31 PM » |
|
Oh great! thanks a lot!
|
|
|
Logged
|
|
|
|
CheeseCakeMan
|
 |
« Reply #909 on: November 24, 2016, 03:48:17 AM » |
|
I've been reading a lot about how to timestep games and the often mentioned Fix Your Timestep! on Gaffer on Games has worked well for me. However I'm currently working on something that has a lot of production buildings and spawners as well as potentially low tickrates for entities far in the distance and I don't quite understand how I would apply this to to things which can only come into existence as a whole.
Stepping physics twice if necessary is fine, we just move half the distance. But...
If I have a spawner with a cooldown of 2 seconds, but I step every 1.1 seconds (exaggerated) I would run the spawner logic after 2.2 seconds for the second time (the time the spawner would answer yes to "Can I spawn?"). To have it spawn every 2 seconds on average I would need to accumulate this time and spawn twice whenever it reaches the cooldown. In the long run it would spawn every 2 seconds, however in this example it would take several seconds for the accumulator to fill and trigger a second spawn. It seems rather odd that all of a sudden two entities appear at the same time, at least from a players perspective.
With amounts this seems doable. A machine could produce 6.4858 kg iron instead of 5 from time to time if the steps don't align. But I can't spawn half a goblin!
|
|
|
Logged
|
|
|
|
ThemsAllTook
|
 |
« Reply #910 on: November 24, 2016, 07:09:56 AM » |
|
Sounds like your spawner entity's tick rate would need to match (or be a multiple of) its cooldown interval. Some time travel might be necessary for things like sounds, which would be more noticeably aliased.
|
|
|
Logged
|
|
|
|
gimymblert
|
 |
« Reply #911 on: November 26, 2016, 09:26:39 PM » |
|
going from cube to sphere without the pinched effect around the pole ... 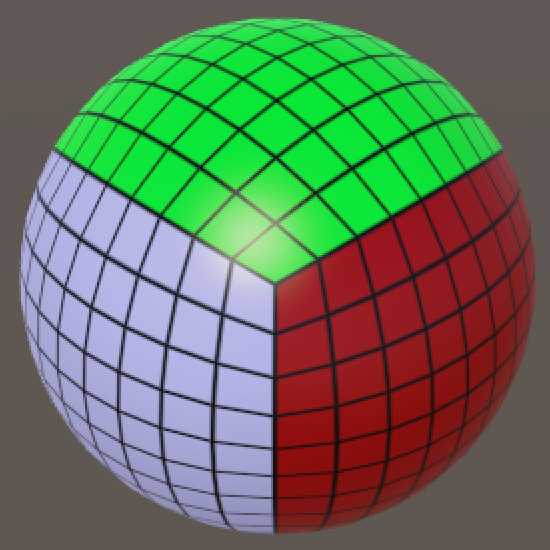 http://mathproofs.blogspot.nl/2005/07/mapping-cube-to-sphere.htmlhttp://catlikecoding.com/unity/tutorials/cube-sphere/http://math.stackexchange.com/questions/118760/can-someone-please-explain-the-cube-to-sphere-mapping-formula-to-meI tried to implement this, Was very excited  as it's a proven method, it should have worked flawlessly ...  x# = VertexX (surface,i) ;*2/grid y# = VertexY (surface,i) ;*2/grid z# = VertexZ (surface,i) ;*2/grid x2# = x*x y2# = y*y z2# = z*z sx# = x * Sqr(1 - (y2 / 2) - (z2 / 2) + ((y2 * z2) / 3)) sy# = y * Sqr(1 - (z2 / 2) - (x2 / 2) + ((x2 * z2) / 3)) sz# = z * Sqr(1 - (x2 / 2) - (y2 / 2) + ((x2 * y2) / 3)) sx# = sx*radius sy# = sy*radius sz# = sz*radius VertexCoords surface, i, sx,sy,sz
why it does not work?  it's the exact same things! (yes I know about the vector.one and grid, I tried) Tell me I'm dumb and I forgot something obvious  I have been staring at this for hours   It's fat but not round Yes I tested the naive version with simply normalizing vertices, it works, BUT I need this smooth non pinched curved.
|
|
« Last Edit: November 26, 2016, 09:42:43 PM by gimymblert »
|
Logged
|
|
|
|
Sik
|
 |
« Reply #912 on: November 27, 2016, 04:50:28 PM » |
|
Looked around at the code I used to generate the globe look-up table in Miniplanets and I think this is the relevant piece of code: float sinx = sin(basex * 3.1415926f * 0.5f); float siny = sin(basey * 3.1415926f * 0.5f); float circx = sinx * sqrt(1.0f - siny * siny * 0.5f); float circy = siny * sqrt(1.0f - sinx * sinx * 0.5f); basex and basey are the original coordinates, circx and circy are the coordinates turned into a circle (values in both cases range from -1 to 1). Shouldn't be hard to add the extra dimension I guess. No guarantees that this is a perfect circle though (and no guarantees that it'll work as intended beyond 90º, but I guess you could just handle it per face instead?), it just happened to work well enough for me. And of course remember to scale the results to whatever range you actually need =P EDIT: in hindsight this does half a sphere when you only want quarter... I guess you should work with coordinates on the -0.5 to +0.5 range then. Take that into account if something goes wrong.
|
|
« Last Edit: November 27, 2016, 05:15:07 PM by Sik »
|
Logged
|
|
|
|
gimymblert
|
 |
« Reply #913 on: November 27, 2016, 10:36:03 PM » |
|
Okay I solved it For some reason, I must multiply by two THEN divide by two  Don't ask the logic, trial and error for the win ...
|
|
|
Logged
|
|
|
|
Thorves
Level 0
|
 |
« Reply #914 on: December 06, 2016, 03:26:42 AM » |
|
In JavaScript: say I have array A and array B. Some of the contents of these arrays may overlap. (e.g. A[0] might be the same object as B[4])
What would be the quickest way to get an array containing all elements in A that are not in B and an array with all elements of B that are not in A?
|
|
|
Logged
|
|
|
|
JohnsyJohnsy
|
 |
« Reply #915 on: December 07, 2016, 03:53:03 AM » |
|
thorves: like so: http://stackoverflow.com/questions/14930516/compare-two-javascript-arrays-and-remove-duplicatesadapted slightly so you dont overwrite your original arrays like this: let arrayA, arrayB, arrayA2, arrayB2
arrayA2 = arrayA.filter(function(val) { return arrayB.indexOf(val) == -1; }); arrayB2 = arrayB.filter(function(val) { return arrayA.indexOf(val) == -1; }); or the es6 way let arrayA, arrayB, arrayA2, arrayB2 arrayA2 = arrayA.filter(val => !arrayB.includes(val)); arrayB2 = arrayB.filter(val => !arrayA.includes(val));
|
|
|
Logged
|
|
|
|
_glitch
Guest
|
 |
« Reply #916 on: December 17, 2016, 01:38:18 PM » |
|
What is the best way to store strings for e.g. dialogs? In a separate class as properties internal static string DiagString { get { return "Some String"; } } Or as var string DiagString = "Some String"; Or directly in the code SpriteBatch.DrawString(Font, "Some String", Position, Color);
|
|
|
Logged
|
|
|
|
Sik
|
 |
« Reply #917 on: December 17, 2016, 05:03:32 PM » |
|
Loaded from a file so it can be easily translated later without even having to touch the code, which means you'll want a different approach altogether (as you'll want a place to keep all of them after loading and the means to fetch them from other parts in the code).
|
|
|
Logged
|
|
|
|
_glitch
Guest
|
 |
« Reply #918 on: December 17, 2016, 11:12:52 PM » |
|
Thank you, I had something like this in mind before.
|
|
|
Logged
|
|
|
|
_glitch
Guest
|
 |
« Reply #919 on: December 18, 2016, 12:13:23 AM » |
|
And what would be the best way to achieve that the right string is read in the file? With using a stream reader? I'm using C#, by the way. 
|
|
|
Logged
|
|
|
|
|